728x90
반응형
조건
1. "가위", "바위", "보" 중 하나를 선택하면 게임 진행
2. "Com:" 옆에 랜덤으로 선택된 결과를 출력하고 "Player:" 옆에 사용자가 선택한 결과를 출력
3.선택한 결과 밑에 게임 진행 결과를 출력
import logo from './logo.svg';
import './App.css';
import { useState } from 'react';
function Title() {
return (
<div className='title'>
<h1>가위 바위 보 게임</h1>
</div>
);
}
function Scissors(props) {
const { onUserChoice } = props;
function run() {
const choice = 'scissors';
console.log(choice);
onUserChoice(choice);
}
return (
<div className='control'>
<img src='http://ggoreb.com/images/react/scissors.png' onClick={run} />
</div>
);
}
function Rock(props) {
const { onUserChoice } = props;
function run() {
const choice = 'rock';
console.log(choice);
onUserChoice(choice);
}
return (
<div className='control'>
<img src='http://ggoreb.com/images/react/rock.png' onClick={run}></img>
</div>
);
}
function Paper(props) {
const { onUserChoice } = props;
function run() {
const choice = 'paper';
console.log(choice);
onUserChoice(choice);
}
return (
<div className='control'>
<img src='http://ggoreb.com/images/react/paper.png' onClick={run}></img>
</div>
);
}
function getRandomChoice() {
const choices = ['scissors', 'rock', 'paper'];
const randomIndex = Math.floor(Math.random() * choices.length);
return choices[randomIndex];
}
function getResultMessage(userChoice, comChoice) {
if (userChoice === comChoice) {
return '비겼습니다!';
} else if (
(userChoice === 'scissors' && comChoice === 'paper') ||
(userChoice === 'rock' && comChoice === 'scissors') ||
(userChoice === 'paper' && comChoice === 'rock')
) {
return '당신이 이겼습니다.';
} else {
return '당신이 졌습니다.';
}
}
function Result(props) {
const [userChoice, setUserChoice] = useState(null);
const comChoice = getRandomChoice();
const resultMessage = getResultMessage(props.userChoice, comChoice);
function handleUserChoice(choice) {
setUserChoice(choice);
}
return (
<div className='result'>
<h1>Com: {comChoice} </h1>
<h1>Player: {props.userChoice || ''} </h1>
<h1>{resultMessage}</h1>
</div>
);
}
function App() {
const [userChoice, setUserChoice] = useState(null);
const handleUserChoice = (choice) => {
console.log(`User chose: ${choice}`);
setUserChoice(choice);
};
return (
<div className="App">
<Title />
<Scissors onUserChoice={handleUserChoice} />
<Rock onUserChoice={handleUserChoice} />
<Paper onUserChoice={handleUserChoice} />
<Result userChoice={userChoice} />
</div>
);
}
export default App;
실행 결과
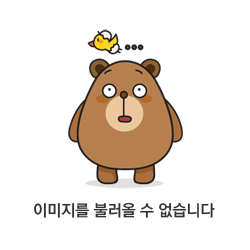
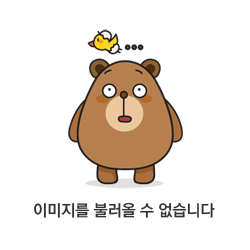
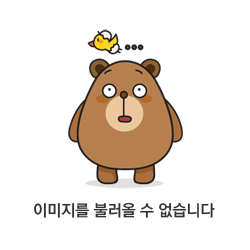
728x90
반응형
'Digital Boot' 카테고리의 다른 글
[Open Weather API] Open Weather로 날씨 API 불러오기 (1) | 2023.11.02 |
---|---|
[Spring Boot] Project - 미니 게임 만들기 (0) | 2023.11.01 |
Project Report (1) | 2023.10.30 |
[Html/Java Scrip] - 최대 공약수, 최소 공배수 구하기, 재귀호출 사용 (0) | 2023.09.01 |
[Java Script] - 거스름 돈 구하기 (0) | 2023.08.31 |